JavaScript Functions in miniApps
Overview
JS functions in miniApps® are strings that represent a JS expression or a sequence of JS statements that are evaluated and executed. The return value is the value of the last executed expression, and its type is specified by the type of the function.
miniApps® JS functions are like separate, totally independent scripts. You can not invoke a JS function from within another.
JS functions can be found in the following miniApps® areas:
Validation Functions for non-intent miniApps
Output Functions for WebService and Intelli miniApps
Types of JS functions
User functions
User functions help do computations and provide an outcome that can be announced or used later as input to another miniApp, and replace an endpoint or a request body in WebService miniApps.
You can use Javascript, write your own code, apply your business logic and at the end literally return a string.
Your javascript function must always return a string in the end.
Example:
A user function that gets the name of the weekday.
const weekday = ["Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday"];
const d = new Date();
//the last expression is the return value and must evaluate to a String
weekday[d.getDay()]; //this will return a value from the array above
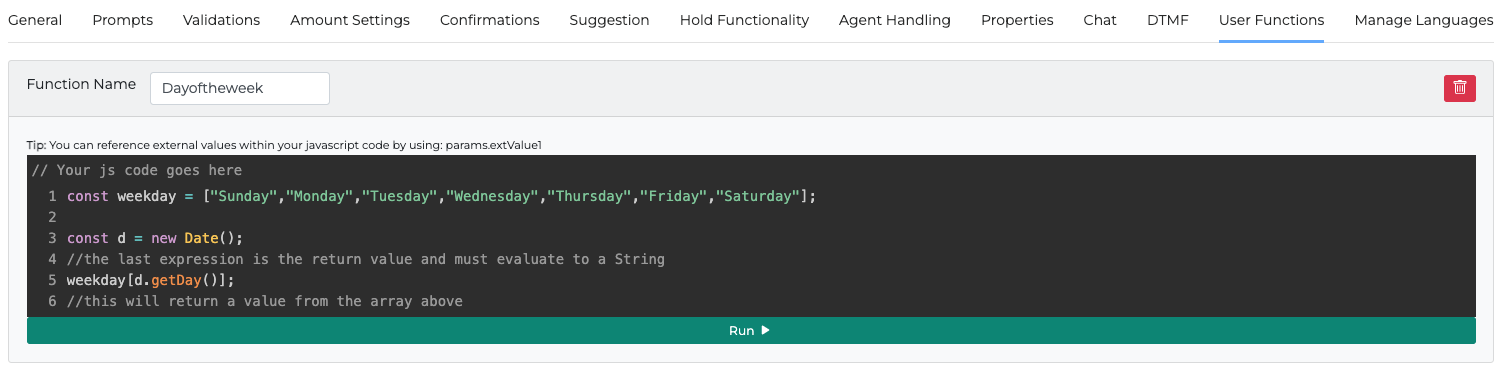
A JS function created under the User Functions tab can be called from everywhere in the specific miniApp, such as prompts in all miniApps, web service settings in Web Service miniApp using the mustache notation {{userFunctionName}}
, or an output path in a Web Service miniApp.
Similar to an output function or a validation function, we don't use the return keyword, but we just end our script with the value to be returned or a reference to it.
However, if we use an inner function, then in the inner function we have to use the return keyword.
In a user function we can take advantage of the params object and at the end, it returns a string value. Even if the output is an integer or an object, the output will be converted to string.
Example:
//calculate localHour utc + 3h
var localHour = parseInt(params.CurrentHour) + 3;
//create greeting prompt
if (localHour < 12) {
"Good Morning";
} else if (localHour < 18) {
"Good Afternoon";
} else {
"Good Evening";
}
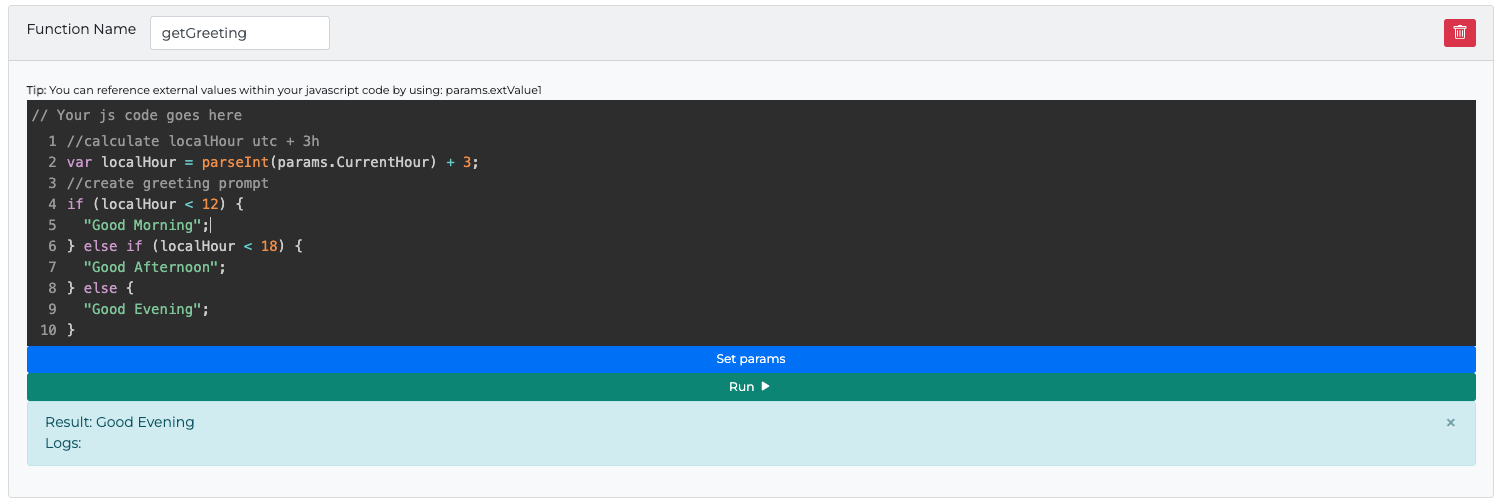
Example with inner function:
function calculateLocalHour(){
// UTC + 3h
var hour = parseInt(params.CurrentHour) + 3;
return hour;
}
var localHour = calculateLocalHour();
//create greeting prompt
var prompt = "";
if (localHour < 12) {
prompt = "Good Morning";
} else if (localHour < 18) {
prompt = "Good Afternoon";
} else {
prompt = "Good Evening";
}
prompt;
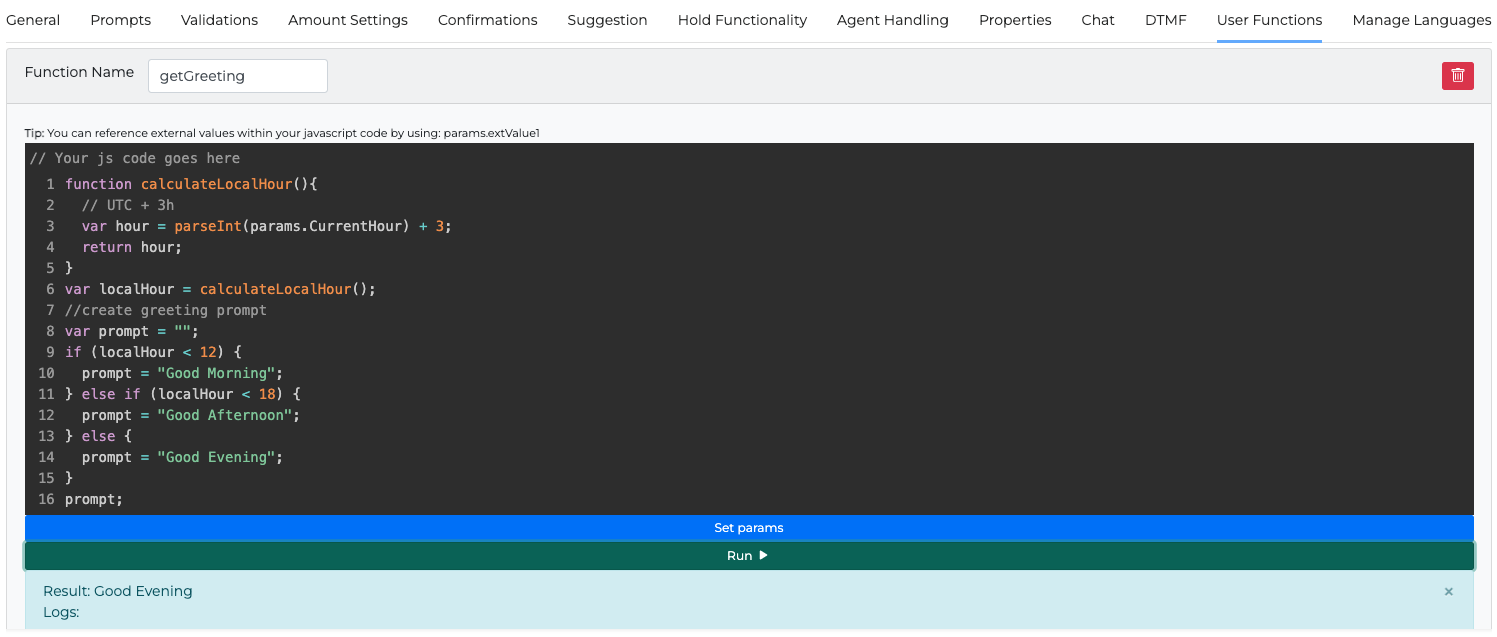
Use case 1: Replace a prompt or a part of a prompt.
Example: {{getGreeting}} -> "Good Morning" or "Good afternoon" or "Good Evening".

Use case 2: Replace the WS endpoint or part of it.
Example: Endpoint: https://example.com/api/filter?timeFrom={{getEpoch}} -> https://example.com/api/filter?timeFrom=1660136594
Validation functions
Validation JavaScript function can be used in the Validations tab in non-Intent miniApps like Alpha, Numeric, Alphanumeric, Amount, Date, and Text.
This feature provides the ability to choose how the data provided by the caller is validated against preset rules.
The validation JS function must always return a boolean in the end.
Use case example: Validate users input using a Javascript function.
In a Validation Function we can take advantage of the params object especially the valueToValidate
property which contains the value of the user's input, and at the end, should return a boolean.
Similar to an Output function or a User function, we don’t use the return keyword but we just end our script with the value to be returned or a reference to it.
Example:
In the Numeric miniApp the caller said “one two three four fifty-six” so the params.valueToValidate
holds the value "123456" . We need to accept numeric values with a length of 6 digits.
if(params.valueToValidate.length == 6) {
true;
} else {
false;
}
or simpler
params.valueToValidate.length == 6;
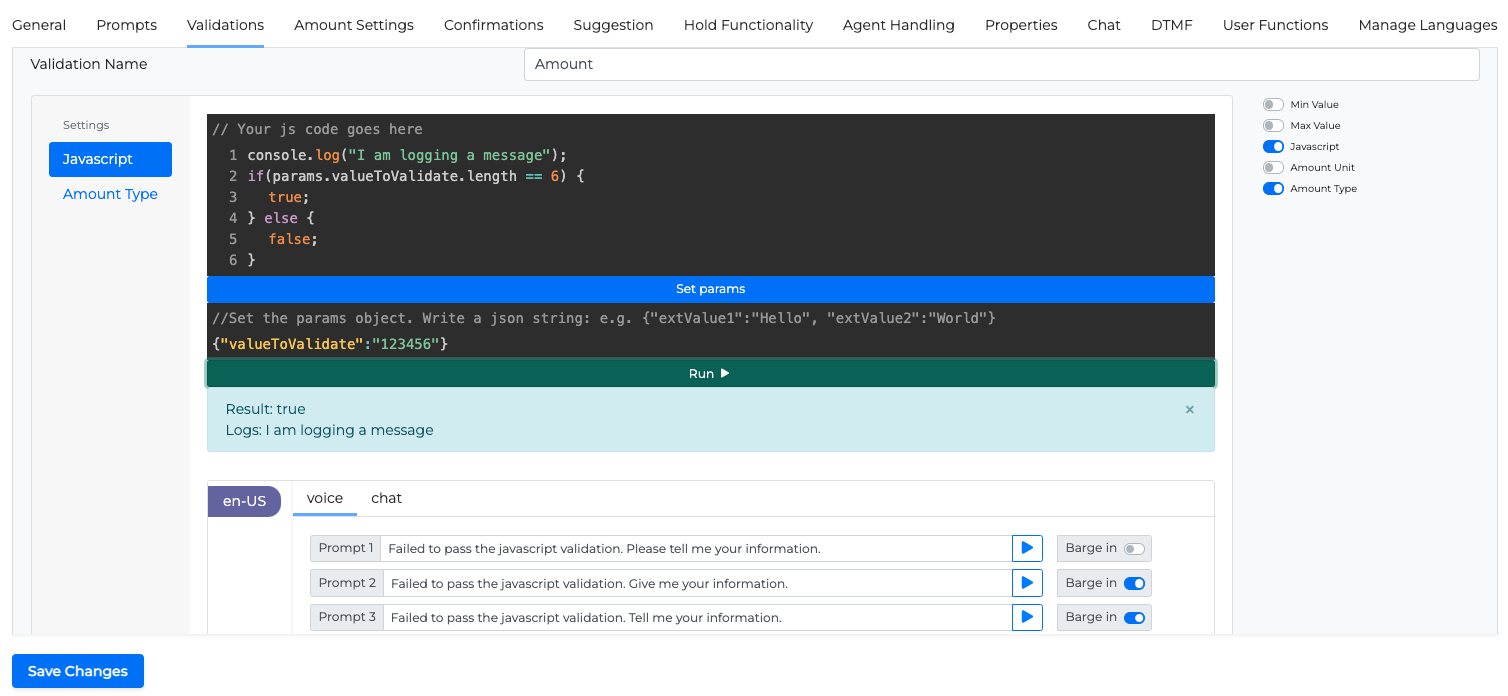
Output functions
The Output function is available under Output tab in Web Service and Intelli miniApps. Web Service or Intelli miniApp are capable of returning multiple string values or JSON objects to our application.
Output function should return an object.
Example:
{
output1: "value1",
output2: {
firstName: "John",
lastName: "Doe",
age: 39
},
output3: "value3",
...
outputN: "valueN"
}
While configuring our miniApp’s inputs and outputs through Orchestrator®, we can select to which application’s field each output will be assigned.
Similar to a User function or a Validation function, we don’t use the return keyword but we just end our script with the object or a reference to it.
However, if we use an inner function, then in the inner function we have to use the return keyword.
Use case example: Parse the output body of a Web Service call and return values to the Orchestrator® app.
Response code from Web Service call: 200
Response body from Web Service call:
{
cardType: “credit”,
cardNetwork: “visa”,
balanceDetails: {
balance: 1234.56,
dueDate: “2022-12-23”,
minimumPayment: 123.45
}
}
Output Function:
var result;
if (params.wsResponseCode == "200") {
result = {
output1: "success",
output2: params.wsResponseBody.balanceDetails.balance,
output3: params.wsResponseBody.balanceDetails.dueDate,
output4: params.wsResponseBody.balanceDetails.minimumPayment,
};
}else {
result = {
output1: "fail",
output2: "unknownValue",
output3: "unknownValue",
output4: "unknownValue"
};
}
result;
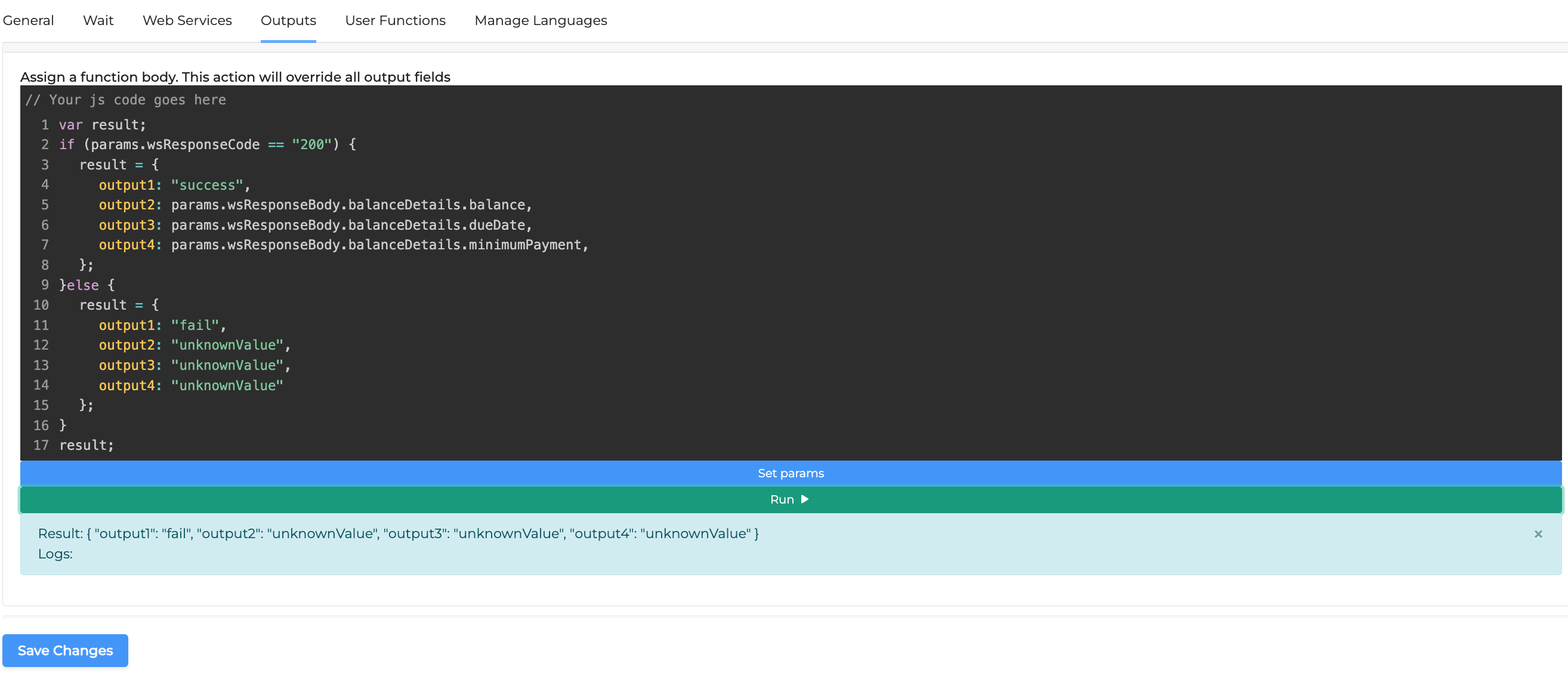
Finally, if you need to consider the Web Service or Intelli miniApp invocations as failed, you can add the FailExitReason
property in the output object like the example below.
var result;
if (params.wsResponseCode == "200") {
result = {
output1: "success",
output2: params.wsResponseBody.balanceDetails.balance,
output3: params.wsResponseBody.balanceDetails.dueDate,
output4: params.wsResponseBody.balanceDetails.minimumPayment,
};
}else {
var errorMessage;
switch (params.wsResponseCode):
case "400":
errorMessage = "BAD_REQUEST";
break;
case "401":
errorMessage = "UNAUTHORIZED";
break;
case "404":
errorMessage = "NOT_FOUND";
break;
case "500":
errorMessage = "INTERNAL_SERVER_ERROR";
break;
default:
errorMessage = "UNKNOWN_ERROR";
break;
result = {
output1: "fail",
output2: "unknownValue",
output3: "unknownValue",
output4: "unknownValue",
FailExitReason: errorMessage
};
}
result;
Node Version
Node.js v20. The ECMAScript compatibility can be found in this table under the 20.17.0 column.
Params Object
To access any of the available properties through a JS function, you need to use the dot (.) notation within the function.
Example:
To get the value of the ANI:
params.Ani
Properties
miniApp details
Property | Description | Example Value |
---|---|---|
| The group the miniApp belongs to | |
| The short name of the miniApp | AskIntent |
| The creator's ID | 1234abc-567d-890e-123f-456789ghijkl |
| The full ID of the miniApp | 1234abc-567d-890e-123f-456789ghijkl.AskIntent.Insurance.MiniApps.groupname |
Session details
Property | Description | Example Value |
---|---|---|
| Automatic Number Identification, the caller’s phone number | |
| Dialed Number Identification Service, the phone number that user called | |
| The session’s locale | en-US |
| IVR Related ID | |
| A common ID for all miniApp invocations of a single dialog | |
| A unique ID for each dialog and miniApp invocation | |
| Turns session in testMode | true |
| The number of the step in dialog | |
| Timestamp in ISO format | 1970-01-01T00:00:00.000 |
| The hour’s value in UTC | 14 |
| The minutes’ value | 59 |
| Number of times the caller asked for a representative within dialog | |
| Number of NoMatch events during dialog | |
| Number of NoInput events during dialog | |
| Total number of errors during dialog | |
| The caller confirmed that they don’t have any further requests | Bot: Your current balance is $12.34. Is there anything else I can do for you? User: No, that will be all |
| Counts the number of times where the user’s input didn’t evolve the dialog | |
| Counts the number of times a user rejected the value in a confirmation step | |
| The value of the input rejected by the user or failed to pass validation | |
| Counts the number of invalid inputs | |
| indicates how the user entered a value | text, DTMF, Voice |
Special properties
Property | Description | Example value |
---|---|---|
| Indicates the failed validation type | preBuiltFailed-CreditCard |
| Indicates whether the validation was successful or not | success/fail |
| Holds the value of the user’s input for non-Intent miniApps. Can be used in validations step if Javascript validation is enabled. | |
| Contains the entire Web Service call response body. Available only in Web Service miniApps | |
| Holds the Web Service call response code. Available only in Web Service miniApps | 200, 400, 404, 500 |
| An array of objects. Each object has a name and a value. |
JS
|
miniApps inputs
Each miniApp can receive input from the parent application (Orchestrator® or directly from the Contact Center).
Inputs can be strings or JSON objects
To access these inputs, one can use the following properties of the params object:
extValue1
extValue2
extValue3
…
extValueN
Logging in JS functions
A developer can log messages within JS functions using the print()
and console.log()
functions.
The logs can be found in miniApp's log file for each distinct invocation.
Do not log sensitive information.
Example:
console.log("I am logging a message");
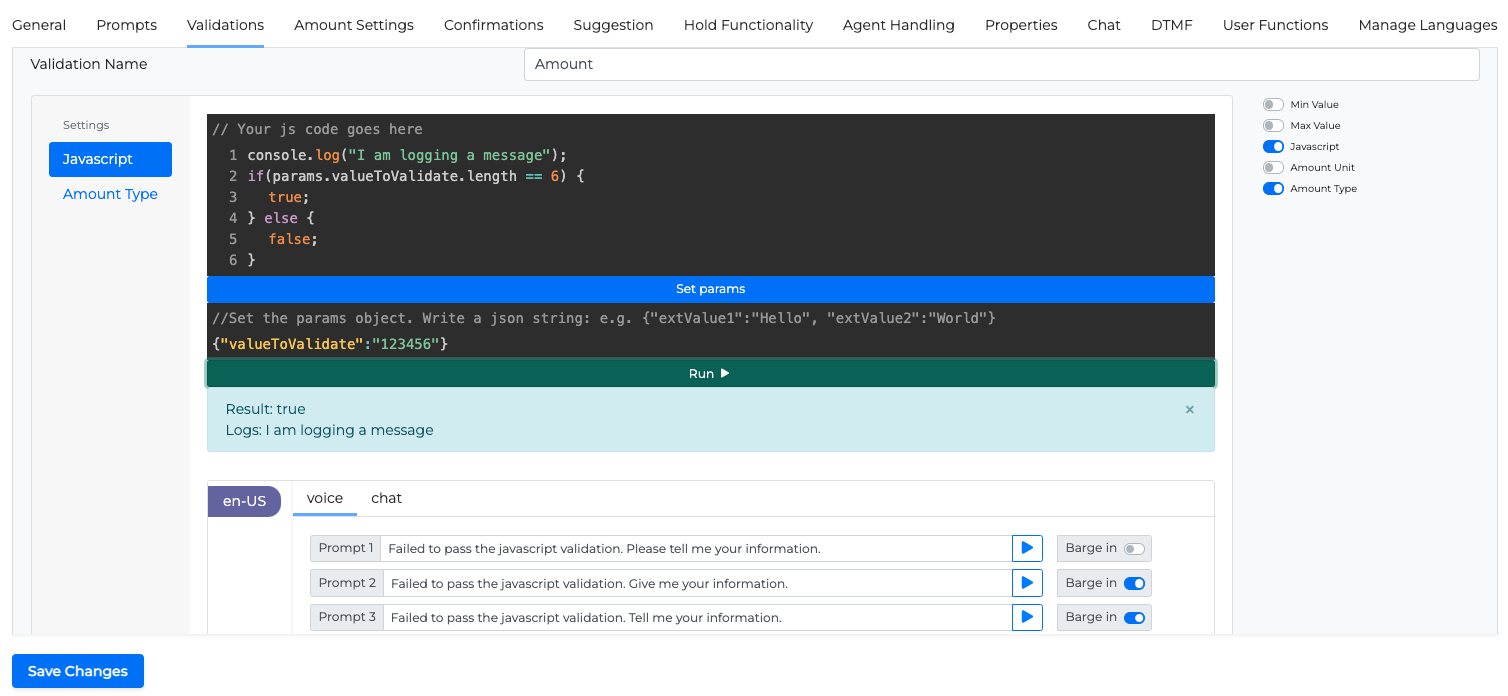
Response Headers display
Response Headers display is available in WebService and Intelli miniApps.
You have the ability to view the response headers, just like the response body, so you can utilize their data.
The application proceeds to send these response headers as an array of objects, each containing a header name and its respective value, to the js-api.
Here's a sample representation of the response headers:
params.wsResponseHeaders = [
{name:"testName1",value:"testValue1"},
{name:"testName2",value:"testValue2"},
{name:"testName2",value:"anotherValueOfTestName2"},
]
This essentially means, for instance, if the response carries the header with the key testName1
and value testValue1, user will be capable of reading this header with the help of js-code.
The below snippet is an illustration of how you can do that:
const valueOfTestHeader = params.wsResponseHeaders.find(header=>header.name==="testName1").value;
console.log(valueOfTestHeader);
"The value of testName1 header is " + valueOfTestHeader
In this scenario, testName1
is the name of the header and the code fetches its corresponding value.
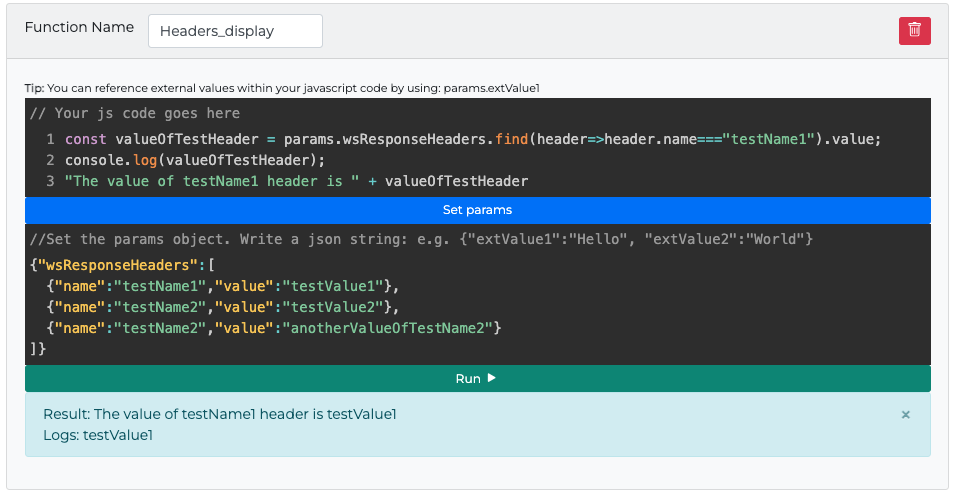
Handlebars Library
Handlebars library is supported for js-api, in both legacy and Isolated modes.
The below snippet is an illustration of how you can do that:
Legacy mode:
// require the library
const handlebars = require("handlebars");
// compile the template
const template = handlebars.compile("Handlebars {{doesWhat}}");
// execute the compiled template
template({ doesWhat: "rocks!" });
Example
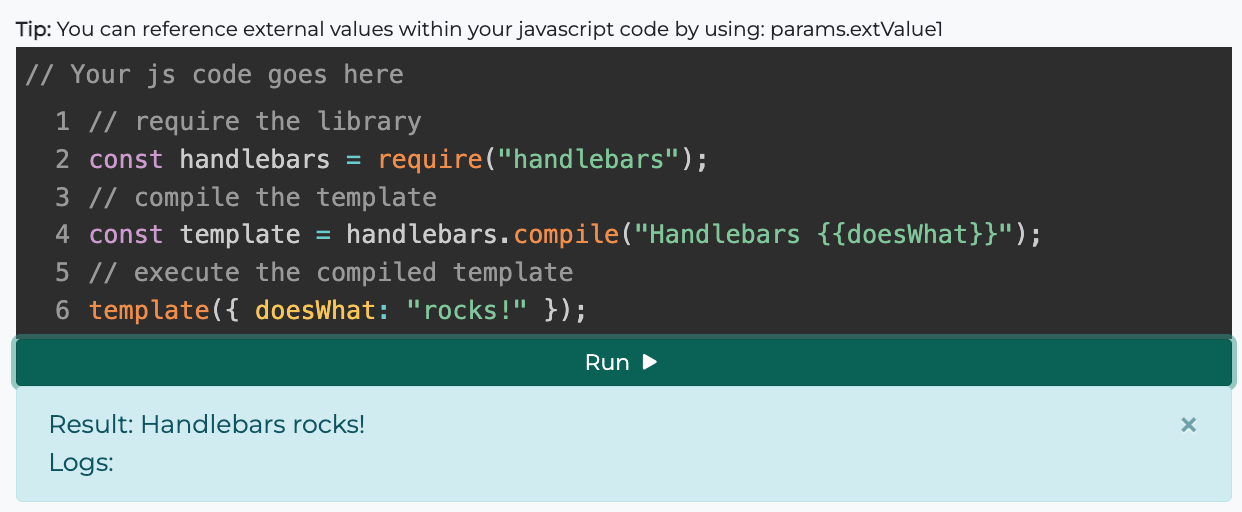
Isolated mode:
// compile the template
const template = Handlebars.compile("Handlebars {{doesWhat}}");
// execute the compiled template
template({ doesWhat: "rocks!" });
Example
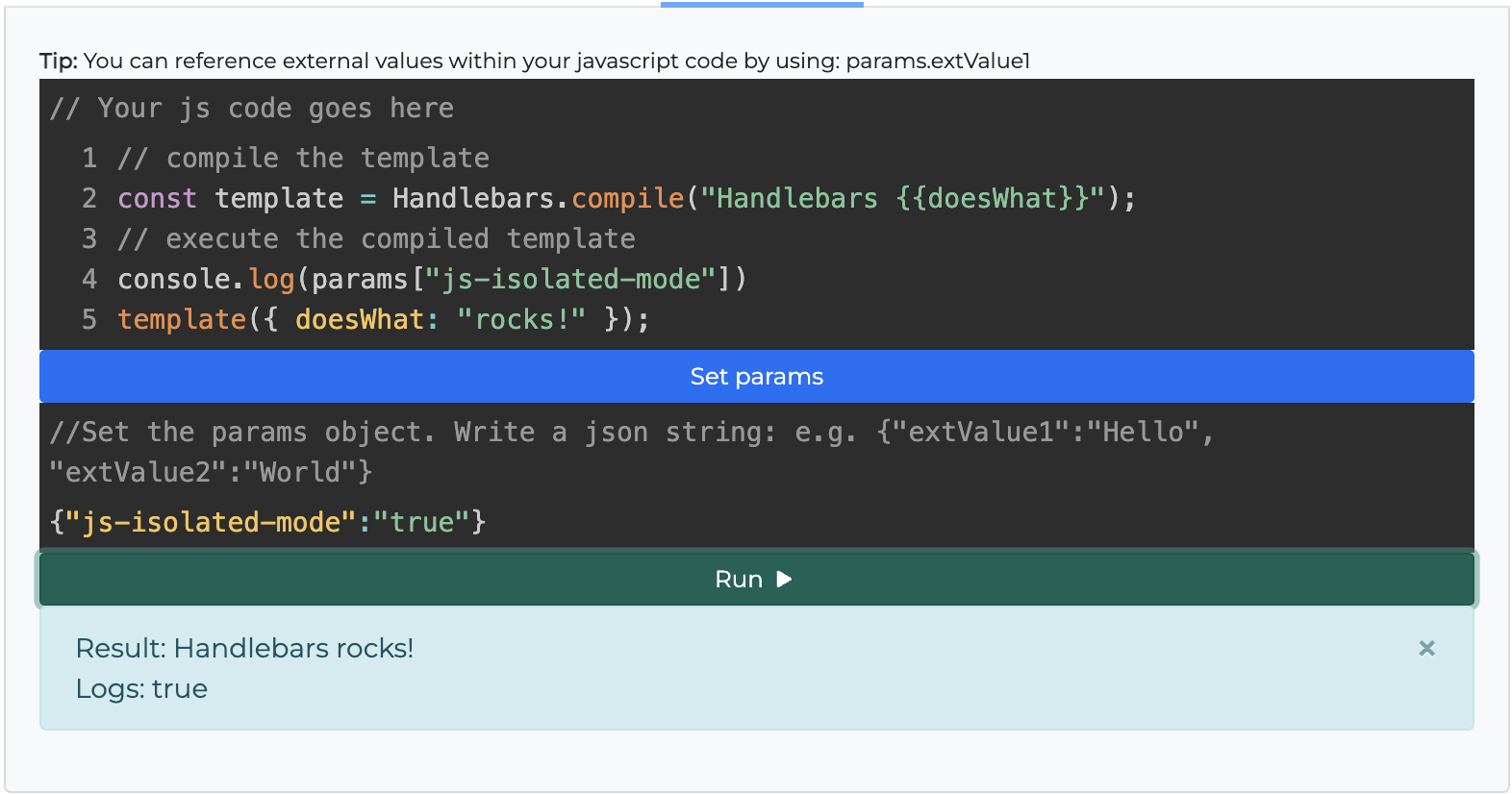
Eta Library
Eta Library is supported for js-api in both legacy and Isolated modes.
The below snippet is an example illustration of how you can do that:
Legacy Mode:
const eta = require("eta");
const etaInstance = new eta.Eta();
etaInstance.renderString("Hello <%= it.name %>", { name: "Ben" })
Isolated Mode:
const etaInstance = new eta.Eta();
etaInstance.renderString("Hello <%= it.name %>", { name: "Ben" })
Testing a JS function
OCP® text editor allows the developer to test a function before even saving it. To do so, write your function, set the additional parameters under the Set params line, and click the Run button.
Example:
{
"valueToValidate":"123456"
}
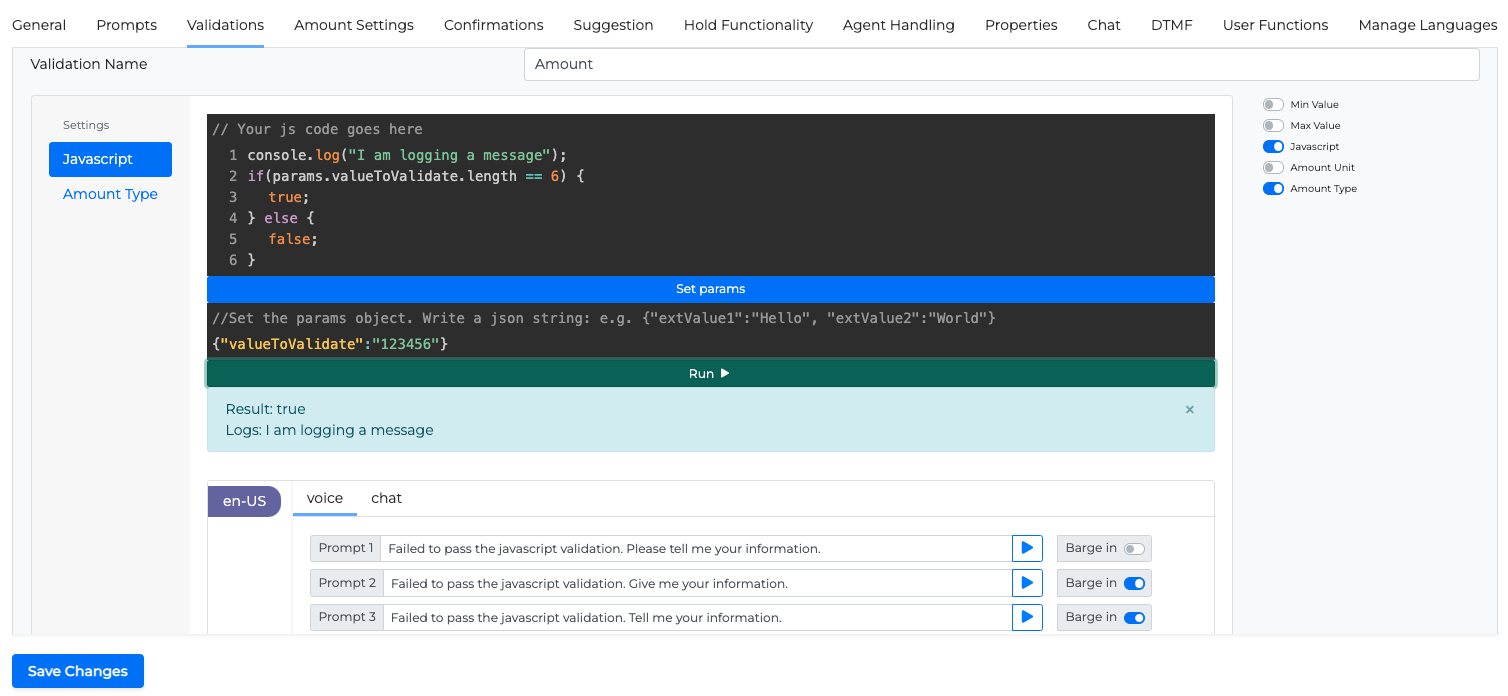
JS API Isolation mode
To improve the security of the JS-API, you can run user scripts in safer, isolated environments. You can select if the JS API will run as usual or select the Isolated Mode.
Legacy Mode: Retaining standard behavior, wherein scripts are executed within a single node environment.
Isolated Mode: Implementing script execution within isolated environments.
The selection of execution mode will be determined by js-isolated-mode
, a flag which can be optionally included in the params object of request through a Set Field in Orchestrator.
A default behavior per environment to handle cases where the flag is absent from the request will be available in later versions.
ocpReturn function
The ocpReturn
function is used to allow users to explicitly define what they want to return from a JS script. The usage of ocpReturn
function ensures that the scripts are executed in isolation mode.
var anObject={}
anObject.a ="A"
anObject.b="B"
ocpReturn(anObject)
This is the recommended method to return a result from JS execution.