Chat API (REST API)
Overview
This documentation outlines the methods for creating and managing messages, attachments, and chat sessions via our REST API. It provides a detailed overview of the available endpoints and the structure of messages sent and received through them.
Authentication
Navigate to the API Authentication page to learn more about Token creation and Authentication process.
Obtaining the API Key Token
Each miniApp has a unique API key token that is essential for establishing communication through the Chat connector.
To find your miniApp's API key token, follow the guidelines below:
Navigate to your OCP → miniApps.
Select a miniApp and click on it to open its settings.
Go to the Chat tab and find the key token within the Webchat Configuration section.
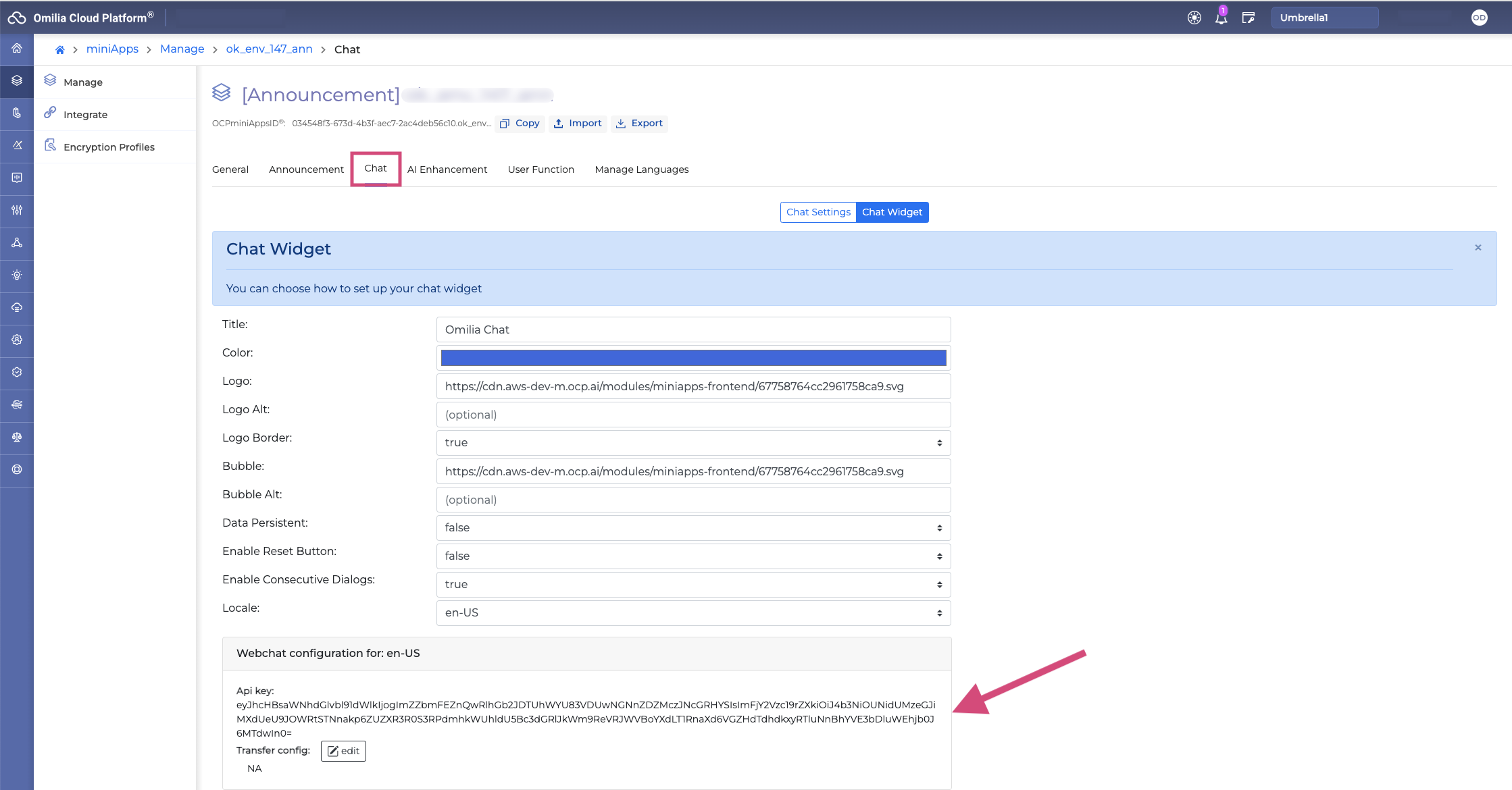
Error Codes
Omilia's HTTP response codes can be found on the HTTP Code Responses page.